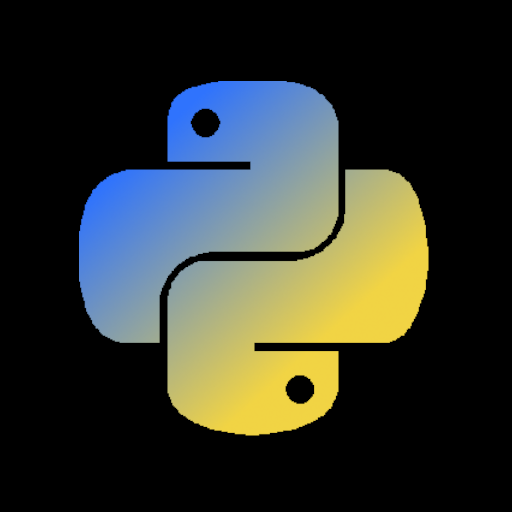
def get_admin_ids(domain: str) -> List[str]:
url = f"https://{domain}/api/v3/site"
response = requests.get(url)
try:
data = response.json()
except json.JSONDecodeError:
print(f"Error: Invalid or empty JSON response for domain {domain}")
return []
admin_ids = [
item["person"]["id"] for item in data["admins"] if item["person"].get("admin")
]
return admin_ids
Conventional Commits